Example: Fugue Notifications in Slack¶
You can integrate Fugue notifications into Slack so that every time Fugue detects compliance, drift, or enforcement events in your environments, a message is sent to Slack.
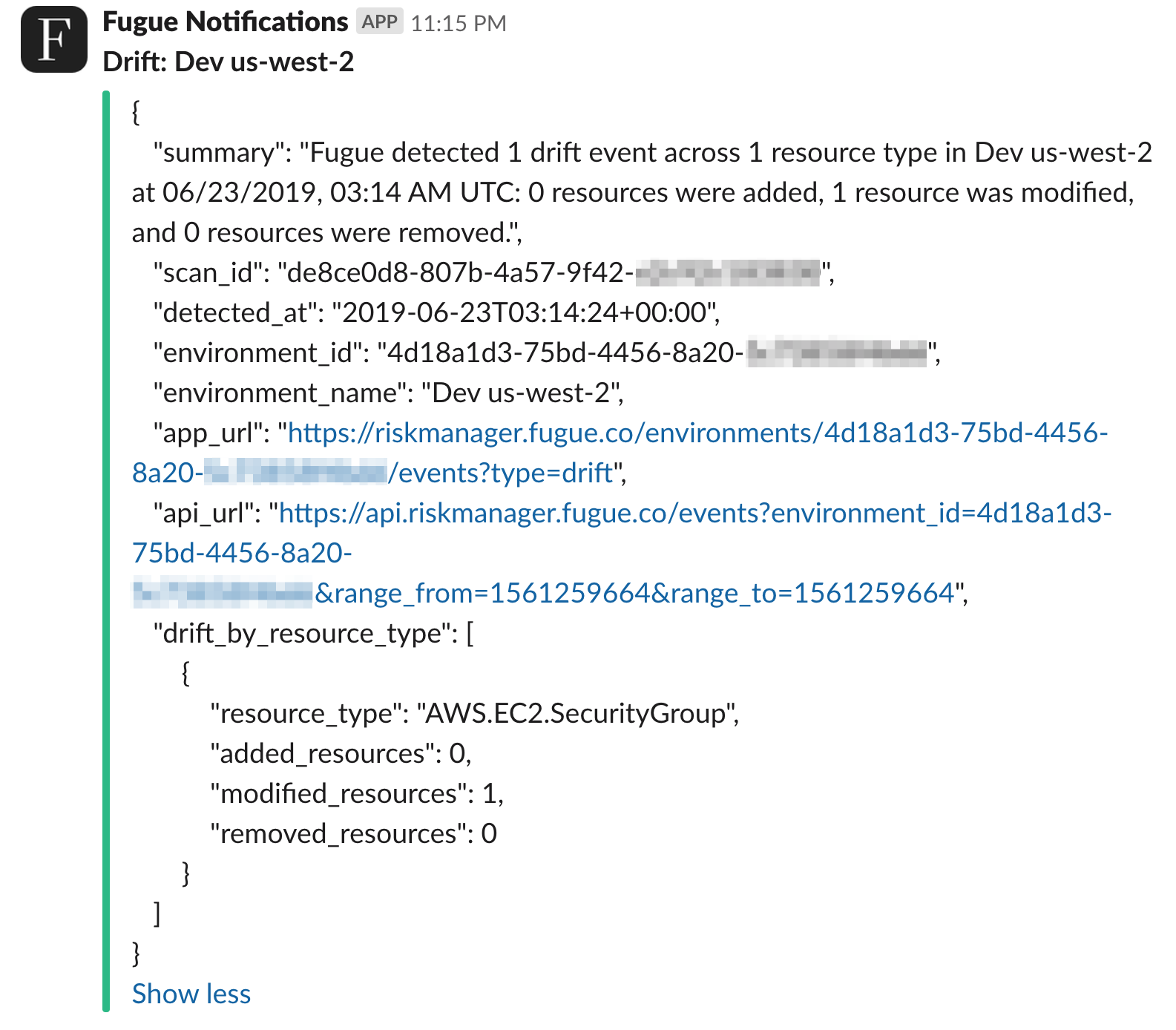
Prerequisite: Create Fugue Notification¶
Note
If you have already set up a notification that you’d like to publish to Slack, skip ahead to Step 1: Create Slack Incoming Webhook.
Create a Fugue notification. Make sure to create an Amazon SNS topic (
FugueSNSTopic
) if you haven’t already and specify its ARN as part of the workflow.
Step 1: Create Slack Incoming Webhook¶
Step 2: Create Lambda Function¶
Log into AWS Lambda Management Console
Select Create function (see Figure A)
Select “Author from Scratch”
Enter function name
Select “Create a new role with basic Lambda permissions”
Select “Create function” button to continue
Paste lambda function code into
index.js
text boxEnter Slack channel in line 9:
'#fugue-notifications'
Enter notification name in line 11:
"*Dev us-west-2 Notifications*"
Copy the webhook URL Slack generated for you in Step 1, starting from /services and paste into line 27:
path: '/services/T02551234/BKG121234/kRg9RPLkdufOzYOT12345678'
’
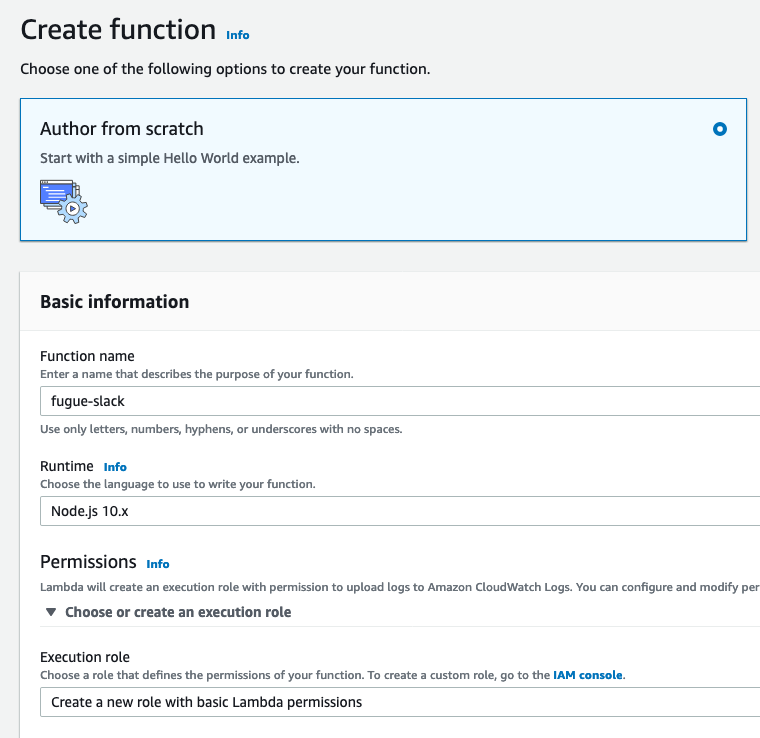
Figure A: Create Lambda function¶
Step 3: Subscribe Lambda Function to FugueSNSTopic¶
Log into Amazon SNS Management Console
Select Subscriptions > Create subscriptions (see Figure B)
Set Topic ARN to
FugueSNSTopic
ARNSet Protocol to AWS Lambda
Set Endpoint to your Lambda function ARN
Select “Create subscription” button to continue
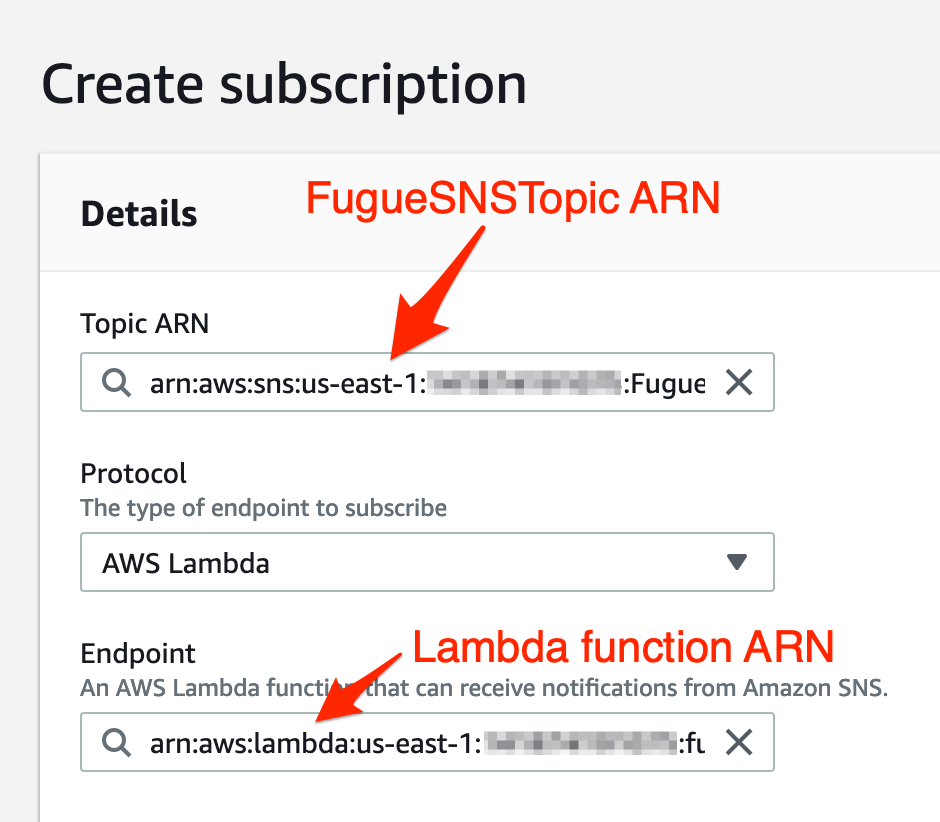
Figure A: Create Lambda function¶
Step 4: Test the Integration¶
Manually make a compliance, drift, or baseline enforcement change in your infrastructure based on how your Fugue notification is configured
Check your Slack channel for a post from the Fugue notification integration
Lambda Function Code¶
Before you paste the following code in AWS Lambda, complete the following steps:
Replace
#fugue-notifications
with your Slack channel (line 9)Replace
*Dev us-west-2 Notifications*
with the name of your notification (line 11)Replace
'/services/T02551234/BKG121234/kRg9RPLkdufOzYOT12345678'
with the incoming webhook path Slack generated for you in Step 1 (line 27)
var https = require('https'); var util = require('util'); exports.handler = function(event, context) { console.log(JSON.stringify(event, null, 2)); console.log('From SNS:', event.Records[0].Sns.Message); var postData = { "channel": "#fugue-notifications", "username": "Fugue Notifications", "text": "*Dev us-west-2 Notifications*", "icon_emoji": ":rotating_light:" }; var message = event.Records[0].Sns.Message; postData.attachments = [ { "text": message } ]; var options = { method: 'POST', hostname: 'hooks.slack.com', port: 443, path: '/services/T02554123/BLP5H1234/7tSULClE1hPYWBjU12345678' }; var req = https.request(options, function(res) { res.setEncoding('utf8'); res.on('data', function (chunk) { context.done(null); }); }); req.on('error', function(e) { console.log('problem with request: ' + e.message); }); req.write(util.format("%j", postData)); req.end(); };
Note
Code and basic instructions adapted from a Medium post from Cohealo.